Notes of a Web Developer Learning Kotlin and Android Development - part 1
Reading time: about 5 minutes
Published
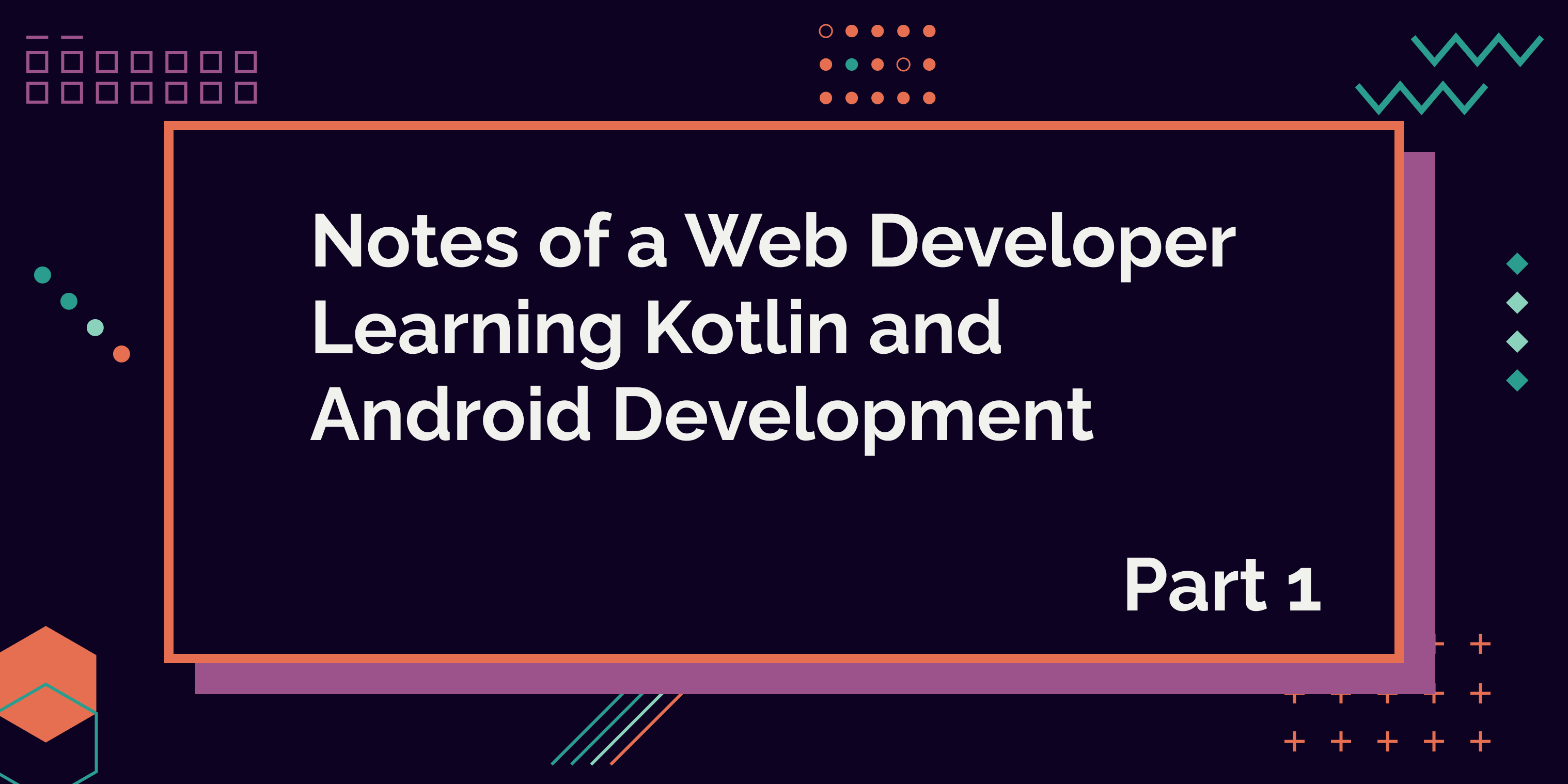
First, a bit of background: I'm an accessibility specialist who recently started digging deeper into our company's Android application. When auditing a web page, it's easy for me to provide technical guidance on how to fix accessibility issues. However, native mobile development and accessibility have been a mystery to me. Now that I've started evaluating our native apps, I've realized I need to understand more from the technical side.
Okay, I have to admit that I'm not a complete newbie in Android development. When I was learning to code about 6-7 years ago, one of the learning paths I was following was Android development. I was working through Google's Android courses in Udacity back then but never finished them.
At the time, the language for Android development was Java, and I've never felt too comfortable with it. I ended up working as a web developer (one of the other learning paths I was taking) and have been able to avoid Java ever since. Fortunately, today Java is more in the background, and Kotlin seems to be the hot thing, so I decided to learn it with Android development.
In this and upcoming blog posts, I will share thoughts, learnings, and reflections from learning Kotlin and Android development. My initial plan is to work through the Android Basics in Kotlin and, after that, learn more about Android accessibility specifically.
Let's start with the first unit (Kotlin Basics). It is about the basics of Kotlin and Android - adding text and images and creating a simple, interactive interface.
First Impressions from Kotlin
I admit I've been a bit prejudiced towards Kotlin because many people have been selling it to me with the idea that it's like "better Java." I've never particularly liked Java.
However, now that I started learning Kotlin, I'm feeling like... Wow, why haven't I tried this before? For me, it resembles Python a bit. I can't exactly pinpoint why, but I get this same soft feeling when coding in Python.
Okay, I do just some dabbling with Python for fun, and I'm rarely working with it, but I really like it. So I can wholeheartedly say that the first impressions from Kotlin were positive.
I know that part of feeling like this is because I've been coding for a long time now, and I've learned at least the basics of many languages. I know JS, TS, Python, Java, Clojure, and Ruby, to name a few. And learning new (programming) languages gets easier after every new language.
Let's look at some concrete learnings and findings from Kotlin from the first unit. I collected the topics from the first unit and did some extra reading to understand some differences and concepts.
Variable Names
The first thing to learn with a new language is usually variables and what's their syntax. I collected three keywords from the first unit to define variables: val
, var
, and const.
val
val
is used to define read-only, local variables.var
var
is used to define mutable variables.const
const
is used to define immutable values (the same as "val"). The difference is that these variables are known at compile time. An example from the course:TAG
was used to define the component name when usingLogger
, and was set toMainActivity
.
Another important keyword is fun
, which defines functions. An example:
fun petACat() {
// ...
}
That is straightforward, as JavaScript has a similar syntax with the function
-keyword. I just need to remember the different naming.
Some Nice Methods and Data Types
The course went through some interesting and useful data types (range
) and methods (random
, when
-statement, and repeat
). I know that most, or all, are present in the other languages I mentioned learning. However, I'm working primarily with JavaScript, so that's what I'm comparing Kotlin to within my mind.
Ranges and Random-Method
One of the apps created on the course was a dice-throwing app. The functionality is simple: a user clicks a button, and the app shows a random number between 1 and 6.
To accomplish this, the code uses a range to create a set of possible values (as the values are in a range between 1 and 6). And that range (IntRange
) has a method called random
, which, as you would guess, returns a random number that is on that range.
It's so much more straightforward than in JavaScript. I like it a lot. Here's an example:
val range = 1..6
val randomNum = range.random()
When - Statement
Another cool method and/or statement is the when
-syntax. I like the way you can, for example, define values with fewer lines of code with it:
val knitGauge10Cm = when(getYarnWeight()) {
"Lace" -> "32-34 sts"
"Fingering" -> "28 sts"
"Sport" -> "24-26 sts"
"DK" -> "22 sts"
"Worsted" -> "20 sts"
"Aran" -> "18 sts"
"Bulky" -> "14-15 sts"
else -> "Unknown"
}
In the example above, when
defines a gauge for 10 cm (or 4 inches) for a knit based on yarn weight. When knitting a garment, it is crucial to know how many stitches there are in a certain width of the knit to match the intended size. So if I had an app helping to calculate the gauge for different types of yarns, this example code would be helpful.
If you're interested in reading more about knitting and gauges, check out Knit Picks' guide about gauge.
Repeat - Method
The third method I want to mention is the repeat
-method. It's so convinient to have a method for repeating something x times. In JavaScript, you naturally can do this - but it takes more lines of code. With Kotlin, you can do that with one function:
fun meow(times: Int) {
repeat(times) {
println("Meow")
}
}
Getting Back to Android Development
As mentioned at the beginning of the post, I was learning about Android Development years ago. Because of that, it was relatively easy to jump back to the world of Android Studio and other things. And as the first unit concentrates on fundamental things, it felt familiar and easy.
However, I recognized some things I need to practice in the upcoming units. For example, it was difficult to understand the whole ConstraintLayout
-concept, and which constraint points to where. I think I will need to think and draw some kind of comparison to CSS layouts to understand the differences.
The next unit will be about layouts, so I'd imagine that will get easier. Also, I'll learn about user input.
Wrapping Up
In this blog post, I shared my initial feelings and thoughts from learning about Kotlin and Android development. It's been fun (pun intended), and I'm looking forward to the following units.
In the next blog post, I will cover the second unit, "Layouts." From the Kotlin side, it consists of things like lists, and from the Android side, different layouts and ScrollView
.
Have you learned native (Android) development lately? What have your experiences been? Or do you have any tips for a web developer learning about Android development?